네트워-크
프로그래머스 코딩 테스트 연습 문제
https://programmers.co.kr/learn/courses/30/lessons/43162
코딩테스트 연습 - 네트워크
네트워크란 컴퓨터 상호 간에 정보를 교환할 수 있도록 연결된 형태를 의미합니다. 예를 들어, 컴퓨터 A와 컴퓨터 B가 직접적으로 연결되어있고, 컴퓨터 B와 컴퓨터 C가 직접적으로 연결되어 있
programmers.co.kr
1. 문제
문제 설명
네트워크란 컴퓨터 상호 간에 정보를 교환할 수 있도록 연결된 형태를 의미합니다. 예를 들어, 컴퓨터 A와 컴퓨터 B가 직접적으로 연결되어있고, 컴퓨터 B와 컴퓨터 C가 직접적으로 연결되어 있을 때 컴퓨터 A와 컴퓨터 C도 간접적으로 연결되어 정보를 교환할 수 있습니다. 따라서 컴퓨터 A, B, C는 모두 같은 네트워크 상에 있다고 할 수 있습니다.
컴퓨터의 개수 n, 연결에 대한 정보가 담긴 2차원 배열 computers가 매개변수로 주어질 때, 네트워크의 개수를 return 하도록 solution 함수를 작성하시오.
제한사항
- 컴퓨터의 개수 n은 1 이상 200 이하인 자연수입니다.
- 각 컴퓨터는 0부터 n-1인 정수로 표현합니다.
- i번 컴퓨터와 j번 컴퓨터가 연결되어 있으면 computers[i][j]를 1로 표현합니다.
- computer[i][i]는 항상 1입니다.
n | computers | return |
3 | [[1, 1, 0], [1, 1, 0], [0, 0, 1]] | 2 |
3 | [[1, 1, 0], [1, 1, 1], [0, 1, 1]] | 1 |
예제 #1
아래와 같이 2개의 네트워크가 있습니다.
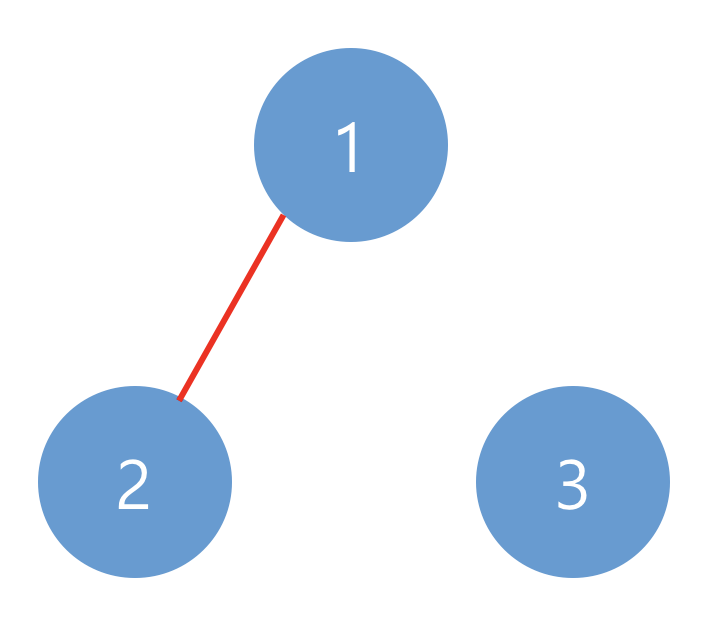
예제 #2
아래와 같이 1개의 네트워크가 있습니다.
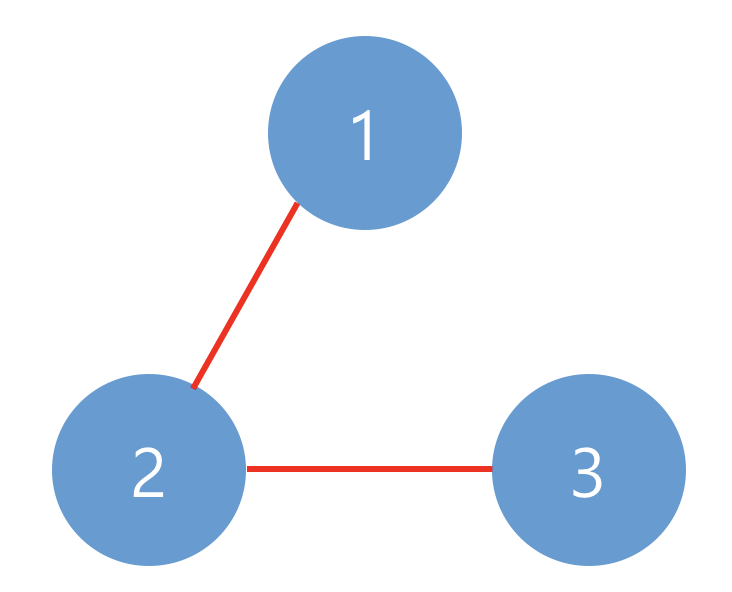
2. 풀이
연결된 노드를 탐색하는 dfs메서드를 만들고 각 노드마다 메서드를 적용(탐색한 노드는 넘어감)해서 연결된 노드의 개수를 카운트한다.
[필드 목록]
boolean[] check;
ArrayList<Integer>[] edge;
int count;
● check : 방문한 노드를 true로 표시
● edge : 인접리스트
● count : 연결된 그래프의 개수를 카운트
[dfs]
public void dfs(int number) {
check[number] = true;
for (int node : edge[number]) {
if (!check[node]) {
dfs(node);
}
}
}
● 해당 메서드에 들어온 것은 매개변수 number의 노드를 방문했다는 의미하기 때문에 number의 인덱스의 check를 true로 변환
● 해당 number의 인접 리스트를 반복문으로 돌며 dfs()를 다시 호출하여 노드를 탐색(이미 방문한 node는 넘어감)
[solution]
public int solution(int n, int[][] computers) {
check = new boolean[n];
edge = new ArrayList[n];
//인접리스트로 변환
for (int i = 0; i < n; i++) {
edge[i] = new ArrayList<Integer>();
for (int j = 0; j < computers[i].length; j++) {
if (i == j) {
continue;
}
if (computers[i][j] == 1) {
edge[i].add(j);
}
}
}
//연결된 노드를 탐색
for (int i = 0; i < n; i++) {
if (check[i]) {
continue;
}
dfs(i);
count++;
}
return count;
}
● 매개변수로 받은 네트워크 관계 배열 computers를 인접 리스트로 변환한다.
◎ 주어진 배열 특성상 자기 자신을 나타내는 i = j 가되는 위치는 넘어간다.
● 각 노드를 출발점으로 dfs()를 사용해서 연결된 노드를 탐색한다.
◎ 탐색된 노드는 넘어가며 이것을 반복하여 연결된 노드를 카운트한다.
[전체 코드]
import java.util.ArrayList;
public class Solution {
boolean[] check;
ArrayList<Integer>[] edge;
int count;
public void dfs(int number) {
check[number] = true;
for (int node : edge[number]) {
if (!check[node]) {
dfs(node);
}
}
}
public int solution(int n, int[][] computers) {
check = new boolean[n];
edge = new ArrayList[n];
for (int i = 0; i < n; i++) {
edge[i] = new ArrayList<Integer>();
for (int j = 0; j < computers[i].length; j++) {
if (i == j) {
continue;
}
if (computers[i][j] == 1) {
edge[i].add(j);
}
}
}
for (int i = 0; i < n; i++) {
if (check[i]) {
continue;
}
dfs(i);
count++;
}
return count;
}
}
'알고리즘 > DFS' 카테고리의 다른 글
Course Schedule (0) | 2022.06.13 |
---|---|
타-겟 넘버 (0) | 2022.04.14 |
댓글